Progress bars are used to show progress of a task. For example, when you are uploading or downloading something from the internet, it is better to show the progress of download/upload to the user.
In android there is a class called ProgressDialog that allows you to create progress bar. In order to do this, you need to instantiate an object of this class. Its syntax is.
In android there is a class called ProgressDialog that allows you to create progress bar. In order to do this, you need to instantiate an object of this class. Its syntax is.
ProgressDialog progress = new ProgressDialog(this);
Now you can set some properties of this dialog. Such as, its style, its text etc.
.progress.setMessage("Downloading Music :) ");progress.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);progress.setIndeterminate(true);
Apart from these methods, there are other methods that are provided by the ProgressDialog class
Sr. No Title and description
1. getMax() This method returns the maximum value of the progress.
2. incrementProgressBy(int diff)
This method increments the progress bar by the difference of value passed as a parameter.
3. setIndeterminate(boolean indeterminate)
This method sets the progress indicator as determinate or indeterminate
4. setMax(int max)
This method sets the maximum value of the progress dialog.
5. setProgress(int value)
This method is used to update the progress dialog with some specific value.
6. show(Context context, CharSequence title, CharSequence message)
This is a static method, used to display progress dialog.
Example
This example demonstrates the horizontal use of the progress dialog which is in fact a progress bar. It display a progress bar on pressing the button.
To experiment with this example, you need to run this on an actual device after developing the application according to the steps below.
Steps Description
1 You will use Android studio to create an Android application under a package com.example.sairamkrishna.myapplication. While creating this project, make sure you Target SDK and Compile With at the latest version of Android SDK to use higher levels of APIs.
2 Modify src/MainActivity.java file to add progress code to display the progress dialog.
3 Modify res/layout/activity_main.xml file to add respective XML code.
4 Run the application and choose a running android device and install the application on it and verify the results.
Following is the content of the modified main activity file src/MainActivity.java.
package com.smr.myapplication;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.Color;
import android.graphics.drawable.BitmapDrawable;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
Button b1;
private ProgressDialog progress;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
b1 = (Button) findViewById(R.id.button2);
}
public void download(View view){
progress=new ProgressDialog(this);
progress.setMessage("Downloading Music");
progress.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progress.setIndeterminate(true);
progress.setProgress(0);
progress.show();
final int totalProgressTime = 100;
final Thread t = new Thread() {
@Override
public void run() {
int jumpTime = 0;
while(jumpTime < totalProgressTime) {
try {
sleep(200);
jumpTime += 5;
progress.setProgress(jumpTime);
}
catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
};
t.start();
}
}
Modify the content of res/layout/activity_main.xml to the following −
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/textView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:textSize="30dp"
android:text="Progress bar" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials Point"
android:id="@+id/textView2"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true"
android:textSize="35dp"
android:textColor="#ff16ff01" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Download"
android:onClick="download"
android:id="@+id/button2"
android:layout_marginLeft="125dp"
android:layout_marginStart="125dp"
android:layout_centerVertical="true" />
</RelativeLayout>
This is the default AndroidManifest.xml−
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.sairamkrishna.myapplication" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Let's try to run your application. We assume, you have connected your actual Android Mobile device with your computer. To run the app from Android studio, open one of your project's activity files and click Run
icon from the toolbar. Before starting your application, Android studio will display following window to select an option where you want to run your Android application.
Select your mobile device as an option and then check your mobile device which will display following screen −
Just press the button to start the Progress bar. After pressing, following screen would appear −
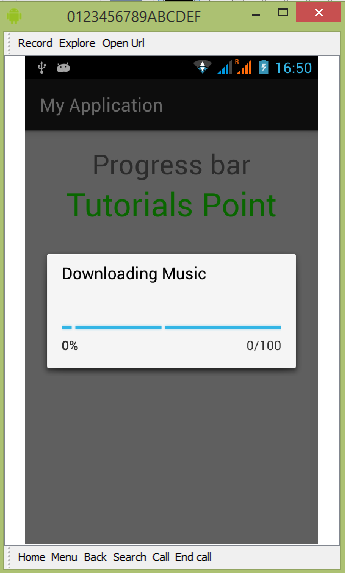
No comments:
Post a Comment